آموزش عملیات حسابی در NumPy
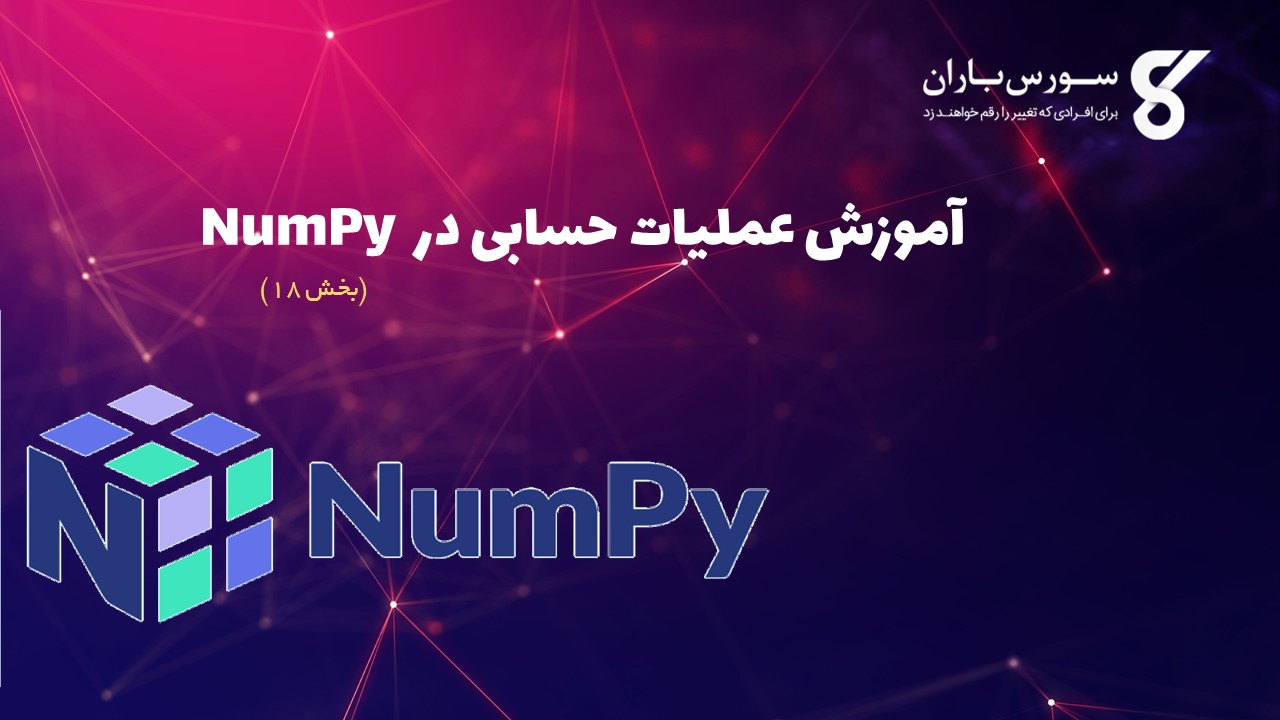
آموزش عملیات حسابی در NumPy
در این درس از مجموعه آموزش برنامه نویسی سایت سورس باران، به آموزش عملیات حسابی در NumPy خواهیم پرداخت.
پیشنهاد ویژه : پکیج آموزش صفر تا صد پایتون
آرایه های ورودی برای انجام عملیات حسابی مانند ()add(), subtract(), multiply, و ()divide باید یک شکل باشند یا باید مطابق با قوانین پخش آرایه باشند.
مثال
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
import numpy as np a = np.arange(9, dtype = np.float_).reshape(3,3) print 'First array:' print a print '\n' print 'Second array:' b = np.array([10,10,10]) print b print '\n' print 'Add the two arrays:' print np.add(a,b) print '\n' print 'Subtract the two arrays:' print np.subtract(a,b) print '\n' print 'Multiply the two arrays:' print np.multiply(a,b) print '\n' print 'Divide the two arrays:' print np.divide(a,b) |
این خروجی زیر را تولید می کند –
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
First array: [[ 0. 1. 2.] [ 3. 4. 5.] [ 6. 7. 8.]] Second array: [10 10 10] Add the two arrays: [[ 10. 11. 12.] [ 13. 14. 15.] [ 16. 17. 18.]] Subtract the two arrays: [[-10. -9. -8.] [ -7. -6. -5.] [ -4. -3. -2.]] Multiply the two arrays: [[ 0. 10. 20.] [ 30. 40. 50.] [ 60. 70. 80.]] Divide the two arrays: [[ 0. 0.1 0.2] [ 0.3 0.4 0.5] [ 0.6 0.7 0.8]] |
اکنون اجازه دهید درباره برخی دیگر از توابع حسابی مهم موجود در NumPy بحث کنیم.
()numpy.reciprocal
این تابع متقابل استدلال را از نظر عناصر برمی گرداند. برای عناصر با مقادیر مطلق بزرگتر از 1 ، نتیجه همیشه 0 است به دلیل روشی که پایتون از تقسیم عدد صحیح استفاده می کند. برای عدد صحیح 0 ، اخطار سرریز صادر می شود.
مثال
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
import numpy as np a = np.array([0.25, 1.33, 1, 0, 100]) print 'Our array is:' print a print '\n' print 'After applying reciprocal function:' print np.reciprocal(a) print '\n' b = np.array([100], dtype = int) print 'The second array is:' print b print '\n' print 'After applying reciprocal function:' print np.reciprocal(b) |
این خروجی زیر را تولید می کند –
1 2 3 4 5 6 7 8 9 10 11 12 13 |
Our array is: [ 0.25 1.33 1. 0. 100. ] After applying reciprocal function: main.py:9: RuntimeWarning: divide by zero encountered in reciprocal print np.reciprocal(a) [ 4. 0.7518797 1. inf 0.01 ] The second array is: [100] After applying reciprocal function: [0] |
()numpy.power
این تابع عناصر موجود در آرایه ورودی اول را به عنوان پایه در نظر گرفته و آنها را به توان عنصر مربوطه در آرایه ورودی دوم برمی گرداند.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
import numpy as np a = np.array([10,100,1000]) print 'Our array is:' print a print '\n' print 'Applying power function:' print np.power(a,2) print '\n' print 'Second array:' b = np.array([1,2,3]) print b print '\n' print 'Applying power function again:' print np.power(a,b) |
این خروجی زیر را تولید می کند –
1 2 3 4 5 6 7 8 9 10 11 |
Our array is: [ 10 100 1000] Applying power function: [ 100 10000 1000000] Second array: [1 2 3] Applying power function again: [ 10 10000 1000000000] |
()numpy.mod
این تابع باقی مانده تقسیم عناصر مربوطه را در آرایه ورودی برمی گرداند. تابع ()numpy.remainder نیز همین نتیجه را ایجاد می کند.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
import numpy as np a = np.array([10,20,30]) b = np.array([3,5,7]) print 'First array:' print a print '\n' print 'Second array:' print b print '\n' print 'Applying mod() function:' print np.mod(a,b) print '\n' print 'Applying remainder() function:' print np.remainder(a,b) |
این خروجی زیر را تولید می کند –
1 2 3 4 5 6 7 8 9 10 11 |
First array: [10 20 30] Second array: [3 5 7] Applying mod() function: [1 0 2] Applying remainder() function: [1 0 2] |
از توابع زیر برای انجام عملیات روی آرایه با اعداد مختلط استفاده می شود.
()numpy.real – قسمت واقعی استدلال نوع داده پیچیده را برمی گرداند.
()numpy.imag – قسمت موهومی استدلال نوع داده پیچیده را برمی گرداند.
()numpy.conj – مزدوج پیچیده را برمی گرداند، که با تغییر علامت قسمت خیالی بدست می آید.
()numpy.angle – زاویه استدلال پیچیده را برمی گرداند. این تابع دارای پارامتر درجه است. در صورت درست بودن، زاویه درجه بازگردانده می شود، در غیر این صورت زاویه در رادیان است.
نسخه ی نمایشی
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
import numpy as np a = np.array([-5.6j, 0.2j, 11. , 1+1j]) print 'Our array is:' print a print '\n' print 'Applying real() function:' print np.real(a) print '\n' print 'Applying imag() function:' print np.imag(a) print '\n' print 'Applying conj() function:' print np.conj(a) print '\n' print 'Applying angle() function:' print np.angle(a) print '\n' print 'Applying angle() function again (result in degrees)' print np.angle(a, deg = True) |
این خروجی زیر را تولید می کند –
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
Our array is: [ 0.-5.6j 0.+0.2j 11.+0.j 1.+1.j ] Applying real() function: [ 0. 0. 11. 1.] Applying imag() function: [-5.6 0.2 0. 1. ] Applying conj() function: [ 0.+5.6j 0.-0.2j 11.-0.j 1.-1.j ] Applying angle() function: [-1.57079633 1.57079633 0. 0.78539816] Applying angle() function again (result in degrees) [-90. 90. 0. 45.] |
لیست جلسات قبل آموزش NumPy
- آموزش NumPy
- معرفی NumPy
- آموزش محیط کار NumPy
- آموزش شی Ndarray در NumPy
- آموزش انواع داده ها در NumPy
- آموزش ویژگی های آرایه در NumPy
- آموزش روال ایجاد آرایه در NumPy
- آموزش ایجاد آرایه از داده های موجود در NumPy
- آموزش ایجاد آرایه از محدوده های عددی در NumPy
- آموزش شاخص گذاری و برش در NumPy
- آموزش شاخص گذاری پیشرفته در NumPy
- آموزش Broadcasting در NumPy
- آموزش تکرار در یک آرایه در NumPy
- آموزش دستکاری آرایه در NumPy
- آموزش اپراتورهای دودویی در NumPy
- آموزش توابع رشته ای در NumPy
- آموزش توابع ریاضی در NumPy
دیدگاه شما